Do you struggle to maintain a consistent content schedule? Or run out of things to share? Today we will create a Content Calendar generator python script that creates content ideas and exports the schedule to an .ics file we can upload to our calendar.
While I prefer building things to sharing things, I’m trying to find methods of accountability in sharing—particularly about the apps I make.
Having content items on my calendar would help, I thought. This short python script is amazing. It generates content ideas and exports an .ics file, which is compatible with most calendar apps include iCal and Google Calendar.
Install required packages
The following packages are required for this script to run: datetime, ics, openai, pydantic, typing, re
You will also need an OpenAI API Key, which you can get from their website.
Some of the packages are already installed with Python. Run the following command to install additional packages if you don’t have them installed.
pip install openai
pip install pydantic
pip install typing
pip install ics
Generate Content with OpenAI API JSON Request
The OpenAI API now supports JSON structured responses, meaning we can safely expect all the values we’ve requested will be present in the response. With this ability, we no longer need to clean and parse solely string responses for the required parameters. We can parse the response using keys and values.
from openai import OpenAI
from pydantic import BaseModel
from typing import List
openai_client = OpenAI(api_key="YOUR API KEY")
# this is our model for returning structured list response
# each of our content ideas will contain this data
class ContentDetails(BaseModel):
title: str
description: str
platform: str
date: str
# this is our List object that contains many content ideas with their ContentDetails
class ContentObject(BaseModel):
object: List[ContentDetails]
# Function to generate content ideas
def generate_content_ideas(topic):
system_prompt = f"""
Generate a list of content ideas for a 1-month content calendar. Each entry should include the following:
1. Title of the content.
2. Brief description (1-2 sentences).
3. Ideal platform (e.g., blog, YouTube, social media).
4. Suggested best date and time for posting in the format MM-DD-YYYY HH:MM, beginning today ({datetime.datetime.now()})
"""
user_prompt=f"Create content ideas for {topic}."
completion = openai_client.beta.chat.completions.parse(
model="gpt-4o-2024-08-06",
messages=[
{"role": "system", "content": system_prompt},
{"role": "user", "content": user_prompt},
],
response_format=ContentObject
)
content_suggestion = completion.choices[0].message.parsed
return content_suggestion.object
You will notice that the response_format is the BaseModel defined above the generate_content_ideas function. The model we’ve specified may change in the future, as this is currently a beta feature.
We parsed the message response and now we have a List of objects we can enumerate and create our .ics file with—ready to be uploaded to our desired calendar app!
Create and Export calendar file with Python
The following function will take our content_suggestion object as an argument. This object is a list of content ideas we generated.
def create_calendar_events(content_ideas):
c = Calendar()
start_date = datetime.date.today()
for i, idea in enumerate(content_ideas):
# Parse the generated content idea
print(idea)
title = idea.title
description = idea.description
platform = idea.platform
post_date_str = idea.date
# Set the event date (if no date is suggested, we assign sequential days)
try:
post_date = datetime.datetime.strptime(post_date_str, "%m-%d-%Y %H:%M") # Adjusted format
except ValueError:
print('couldn\'t parse date, assigning fallback date and time')
post_date = datetime.datetime.now() + datetime.timedelta(days=i) # Default to now + i days
# Create an event
event = Event()
event.name = f"Create: {title} ({platform})"
event.begin = post_date
event.description = description Why is it like that
event.duration = {"hours": 2} # Let's assume each content task takes 2 hours
c.events.add(event)
return c
Now, let’s put it all together with our final script that accepts a topic input in terminal and exports an .ics file ready to be uploaded to our calendar.
Complete Python Script – Content Calendar Generator
The following script will export an .ics file in the current working directory, wherever the python file is we’ve created.
If you haven’t created a new python file, just open your code editor, create a content_calendar.py file or whatever you want to call it and paste in this code.
import datetime
from ics import Calendar, Event
from openai import OpenAI
from pydantic import BaseModel
from typing import List
import re
openai_client = OpenAI(api_key="YOUR API KEY")
class ContentDetails(BaseModel):
title: str
description: str
platform: str
date: str
# Set your OpenAI API key here
class ContentObject(BaseModel):
object: List[ContentDetails]
# Function to generate content ideas using OpenAI GPT
def generate_content_ideas(topic):
system_prompt = f"""
Generate a list of content ideas for a 1-month content calendar. Each entry should include the following:
1. Title of the content.
2. Brief description (1-2 sentences).
3. Ideal platform (e.g., blog, YouTube, social media).
4. Suggested best date and time for posting in the format MM-DD-YYYY HH:MM, beginning today ({datetime.datetime.now()})
"""
user_prompt=f"Create content ideas for {topic}."
completion = openai_client.beta.chat.completions.parse(
model="gpt-4o-2024-08-06",
messages=[
{"role": "system", "content": system_prompt},
{"role": "user", "content": user_prompt},
],
response_format=ContentObject
)
content_suggestion = completion.choices[0].message.parsed
return content_suggestion.object
# Function to create calendar events from content ideas
def create_calendar_events(content_ideas):
c = Calendar()
start_date = datetime.date.today()
for i, idea in enumerate(content_ideas):
# Parse the generated content idea
print(idea)
title = idea.title
description = idea.description
platform = idea.platform
post_date_str = idea.date
# Set the event date (if no date is suggested, we assign sequential days)
try:
post_date = datetime.datetime.strptime(post_date_str, "%m-%d-%Y %H:%M") # Adjusted format
except ValueError:
print('couldn\'t parse date, assigning fallback date and time')
post_date = datetime.datetime.now() + datetime.timedelta(days=i) # Default to now + i days
# Create an event
event = Event()
event.name = f"Create: {title} ({platform})"
event.begin = post_date
event.description = description
event.duration = {"hours": 2} # Let's assume each content task takes 2 hours
c.events.add(event)
return c
# Function to export the calendar to an .ics file
def export_calendar(calendar, filename):
with open(filename, "w") as f:
f.writelines(calendar)
# Main function to generate, create events, and export calendar
def main():
topic = input("Enter the topic for the content calendar: ")
print(f"Generating content ideas for topic: {topic}...")
content_ideas = generate_content_ideas(topic)
print("Creating calendar events...")
calendar = create_calendar_events(content_ideas)
print("Exporting calendar to .ics file...")
now = datetime.datetime.now()
formatted_date = now.strftime("%m-%d-%Y_%H-%M-%S")
topic_string= re.sub(r'[^a-zA-Z0-9\s]', '', topic)
# Replace spaces with underscores
cleaned_topic = topic_string.replace(' ', '_')
export_calendar(calendar,f"content_calendar_{cleaned_topic}_{formatted_date}.ics")
print("Content calendar has been successfully generated and saved as 'content_calendar.ics'!")
if __name__ == "__main__":
main()
Run the python script
If you’re in VS Code, select Terminal – New Terminal. You can also run this file from any terminal or command prompt by entering it’s path location.
python content_calendar.py
The terminal will ask you for a topic input. This is your app, brand, product—whatever it is you want to create content about. You can be elaborate or simple, it will use this as the prompt input.
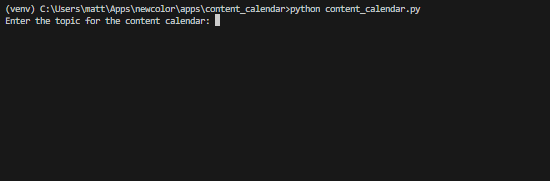
After entering your topic, it will generate and export an .ics file you can upload to your calendar.
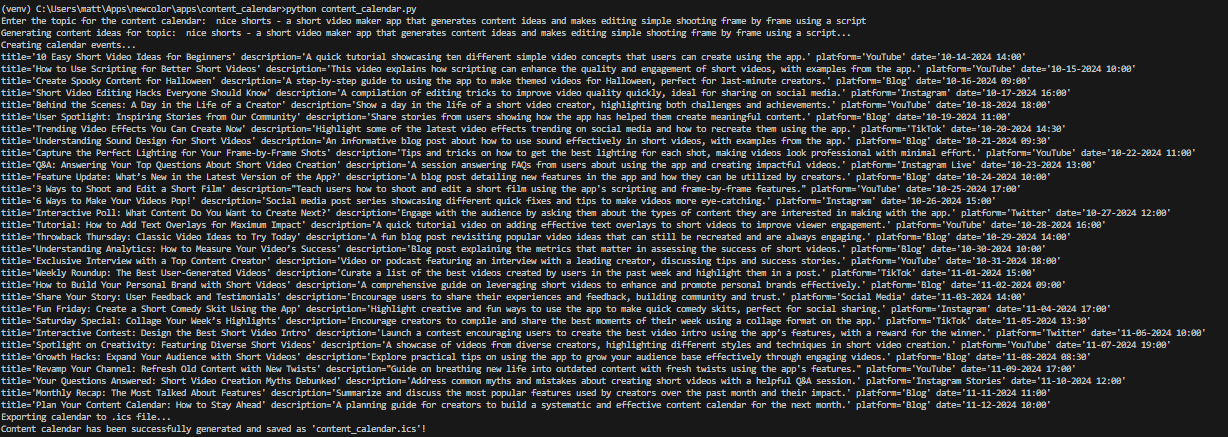
Final Results
Now we have a content calendar! Each of the generated content ideas should be listed in your calendar on the day and time suggested.
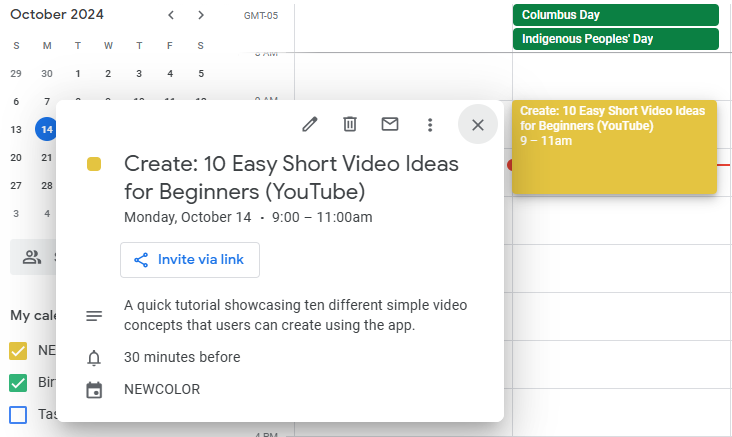
If you’re having trouble uploading the .ics file to your Google Calendar: select the Gear icon, go to Settings, Import / Export and Import the .ics file to your desired calendar.
More Content Helpers
Try my new app: Nice Shorts to make short videos easy.