Spark AR Scripting
Sending variables between the Patch Editor and script is useful in a number of cases. Sometimes, it’s more efficient to run a function in JavaScript than in the Patch Editor. In other cases, it’s the only way to run a particular function.
Download the Free Template
The Spark AR Scripting example project demonstrates how to send and receive all possible variable types: Number, Text, Boolean, Pulse, Vector2, Vector3 and Vector4 values.
The template builds on this post, which contains script snippets for To Script From Script Patches functions.
The primary module used for script to patch bridging is the Patches module. To include the Patches module, place this line at the top of your script:
const Patches = require('Patches');
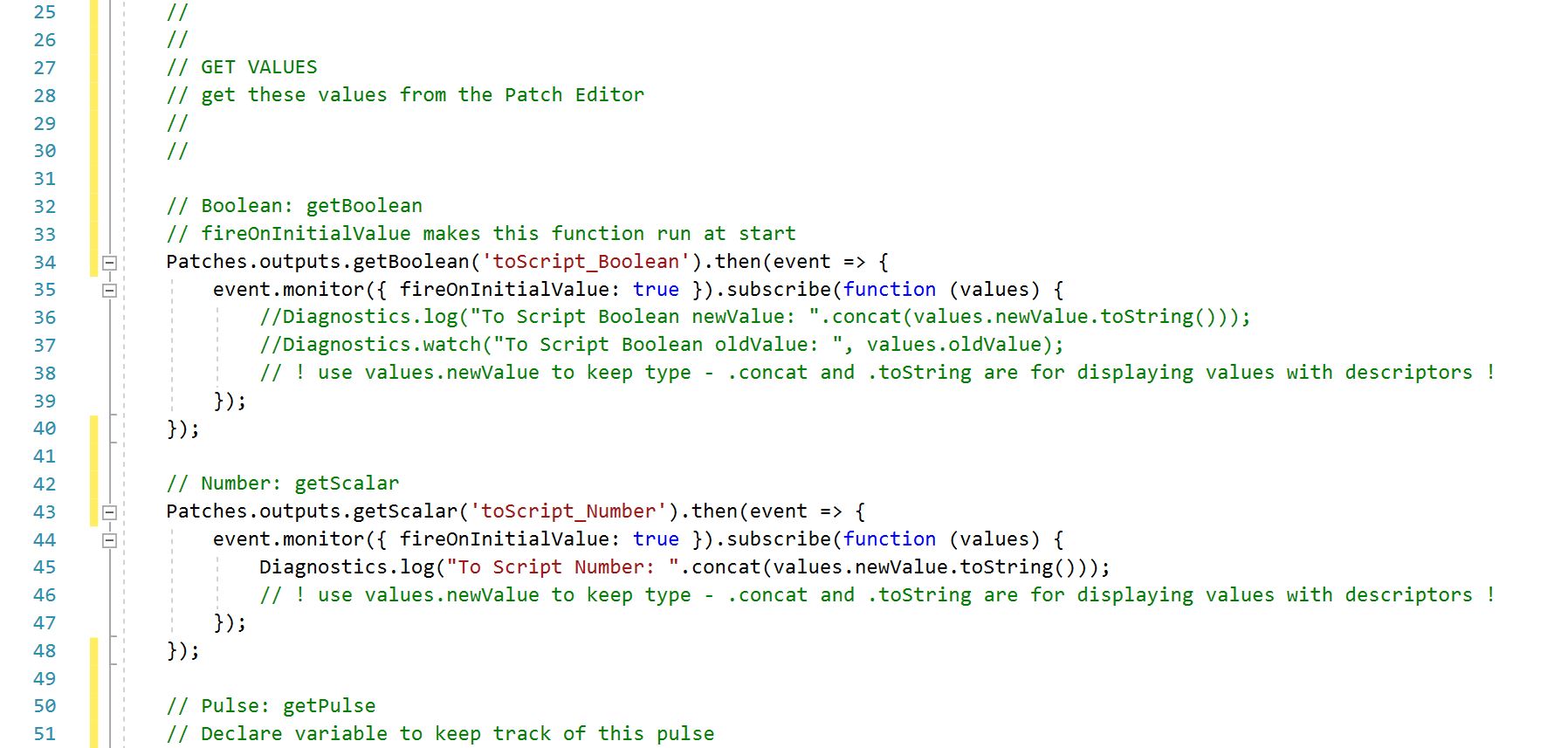
Script to Patch Bridging
To Script
Number: getScalar
Patches.outputs.getScalar('ToScriptNumber').then(event=> {
event.monitor().subscribe(function (values) {
Diagnostics.log(values.newValue);
});
});
Text: getString
Patches.outputs.getString('ToScriptText').then(event => {
Diagnostics.log(event.pinLastValue());
});
Boolean: getBoolean
Patches.outputs.getBoolean('ToScriptBoolean').then(event => {
event.monitor().subscribe(function (values) {
Diagnostics.log(values.newValue);
Diagnostics.log(values.oldValue);
});
});
Pulse: getPulse
// Declare variable to keep track of this pulse
var pulsed;
Patches.outputs.getPulse('ToScriptPulse').then(event => {
pulsed = event.subscribe(function () {
// code to execute when pulse happens
// "pulsed" variable will be available throughout the script
});
});
Vector 2: getPoint2D
// Declare variable with x and y properties
var vector2 = {x: 0, y: 0};
//Vector 2
Patches.outputs.getPoint2D('ToScriptVector2').then(pixelPointSignal => {
// Pin
vector2.x = pixelPointSignal.x.pinLastValue();
vector2.y = pixelPointSignal.y.pinLastValue();
// Monitor
pixelPointSignal.x.monitor({ fireOnInitialValue: true }).subscribe(function (xSignal) {
vector2.x = xSignal.newValue;
});
pixelPointSignal.y.monitor({ fireOnInitialValue: true }).subscribe(function (ySignal) {
vector2.y = ySignal.newValue;
});
});
Vector 3: getPoint
// Declare variable with x, y, z properties
var vector3 = { x: 0, y: 0, z: 0 };
// Vector 3
Patches.outputs.getPoint('ToScriptVector3').then(pointSignal => {
// Pin
vector3.x = pointSignal.x.pinLastValue();
vector3.y = pointSignal.y.pinLastValue();
vector3.z = pointSignal.z.pinLastValue();
// Monitor
pointSignal.x.monitor({ fireOnInitialValue: true }).subscribe(function (xSignal) {
vector3.x = xSignal.newValue;
});
pointSignal.y.monitor({ fireOnInitialValue: true }).subscribe(function (ySignal) {
vector3.y = ySignal.newValue;
});
pointSignal.z.monitor({ fireOnInitialValue: true }).subscribe(function (zSignal) {
vector3.z = zSignal.newValue;
});
});
Vector 4: getColor
// Declare variable with x, y, z, w properties
var vector4 = {x: 0, y: 0, z: 0, w: 0};
// Vector 4
// Cannot monitor this signal without crashing program.
Patches.outputs.getColor('ToScriptVector4').then(colorSignal => {
vector4.x = colorSignal.red.pinLastValue();
vector4.y = colorSignal.green.pinLastValue();
vector4.z = colorSignal.blue.pinLastValue();
vector4.w = colorSignal.alpha.pinLastValue();
});
From Script
Number: setScalar
// Example number to send to Patch Editor from script
var scriptNumber;
Patches.inputs.setScalar('FromScriptNumber', scriptNumber);
Text: setString
// Example string (text) to send to Patch Editor from script
var scriptString = "Example Text";
var scriptNumber = 3;
Patches.inputs.setString('FromScriptText', scriptString);
Patches.inputs.setString('FromScriptNumberAsText', scriptNumber.toString());
Boolean: setBoolean
Patches.inputs.setBoolean('FromScriptBoolean', true);
Pulse: setPulse
// Call this function to pulse 'FromScriptPulse'
// Reactive.once() will send an event pulse
// If it's not in a function, it will fire once on load
function sendPulse() {
Patches.inputs.setPulse('FromScriptPulse', Reactive.once());
}
Vector 2: setPoint2D
// Declare variable to send 2 values
var pixelSignal = Reactive.point2d(10, 20);
// Set the vector 2
Patches.inputs.setPoint2D('FromScriptVector2', pixelSignal);
Vector 3: setPoint
// Declare variable to send 3 values
var pointSignal = Reactive.point(10, 20, 30);
// Set the vector 3
Patches.inputs.setPoint('FromScriptVector3', pointSignal)
Vector 4: setColor
// Vector 4: setColor
// RGBA uses values between 0.0 and 1.0
var point4DSignal = Reactive.RGBA(0.1, 0.2, 0.3, 1);
// Set the vector 4
Patches.inputs.setColor('fromScript_Vector4', point4DSignal)
If you need more Spark AR Scripting support for this template or a custom filter project, send me a message! You can also follow my YouTube channel to learn more about Spark AR Studio topics.